FAQ
Single-page apps (SPA) #
These are also called Progressive Web Apps (PWA) and generally load the site as a single page, rather than loading individual pages as normal.
When a page is loaded for the first time, the Clerk.js library automatically fires a function to render all Content blocks on that page.
However, for single-page apps using frameworks like vue.js or next.js, pages are rendered with JavaScript rather than a standard page load. Due to this, you need to control the rendering with Clerk.js to match how you load pages in the app.
Include Clerk.js #
Clerk.js only needs to be loaded once, when the site is initially loaded. After this, the library will be available throughout the session. Include it just before the </head>
in your HTML:
<!-- Start of Clerk.io E-commerce Personalisation tool - www.clerk.io -->
<script type="text/javascript">
(function(w,d){
var e=d.createElement('script');e.type='text/javascript';e.async=true;
e.src='https://cdn.clerk.io/clerk.js';
var s=d.getElementsByTagName('script')[0];s.parentNode.insertBefore(e,s);
w.__clerk_q=w.__clerk_q||[];w.Clerk=w.Clerk||function(){w.__clerk_q.push(arguments)};
})(window,document);
Clerk('config', {
key: 'INSERT_PUBLIC_API_KEY'
});
</script>
<!-- End of Clerk.io E-commerce Personalisation tool - www.clerk.io -->
Control Rendering #
By default, Clerk.js renders any elements that have the class clerk
, regardless of whether it’s inserted during initial page load or when the DOM mutates. You can control the timing by inserting the element when it’s ready to be rendered.
Alternatively, you can control rendering with the function Clerk("content", "SELECTOR")
.
Every time a page is loaded, do these steps in order:
- Add the Clerk snippet to the HTML with a unique selector you can target.
- Run
Clerk("content", "SELECTOR")
to render it.
When the visitor leaves the page, destroy the snippet, and render it again if the visitor returns to the same page. This is to ensure Clerk.js does not see the snippet as previously rendered, causing it to not visualize.
Example:
<span
id="clerk-custom-snippet"
data-template="@home-page-visitor-recommendations">
</span>
<script>
Clerk("content", "#clerk-custom-snippet")
</script>
Clerk.js can also be configured to automatically initialize Content with your custom selectors after the first time you render it.
Impact on Pagespeed #
Adding an external tool like Clerk.js will increase the time it takes to load your site, but it is negligible compared to the additional conversions it will provide. Below you can see how it impacts your site’s performance.
Performance #
The Clerk.js library is only 37.5kb in size, so it loads very quickly. Also, it loads elements asynchronously, which means that the rest of the page loads while Clerk.js renders content.
An increase in the load time of a page most often comes from loading more images than previously, since Clerk’s search results and recommendations work best when they are visually appealing.
To minimize the extra load time, we recommend using images in webp
format that have a resolution matching the size they have in the Clerk elements.
For example, if images in recommendations have a resolution of 400x400px
in desktop view, send images in a resolution of maximum 420x420px
or similar.
Google Page Speed #
If you use Google Page Speed Insights or a similar tool to analyze your site’s performance, you might see Clerk.js listed under Leverage browser caching.

The purpose of Clerk.js is to make it super simple to insert results from Clerk.io into any website. Clerk.js contains many features to handle tracking and UI components such as Instant Search, sliders, popups and more.
When we add new UI features or make improvements to existing ones, they are included in Clerk.js and must be downloaded by the end user in order to use them.
Having a cache expiry of 60 minutes means that when we release new features they will be available to everyone within a maximum of 60 minutes. The longer the cache time, the longer it takes for everyone to have access to the newest features.
The important thing is that end users only have to download Clerk.js once when new features are available.
The 60 minute cache expiry just means that the end user’s browser will check in with Clerk.io every 60 minutes. If no changes have been made to Clerk.js, nothing will be downloaded.
The cache expiry time of 60 minutes is thus a tradeoff between minimizing web requests and seeping new features and improvements. Most sessions are shorter than 60 minutes and so the request will only be made once per session.
As you can see in the screenshot, this is a normal practice that (as well as Clerk.io) is used by Google Analytics, Facebook, Trustpilot and many others.
CLS Impact #
Cumulative Layout Shift (CLS) can negatively impact SEO and user experience when dynamically injected content shifts elements on a page. In some cases, among other factors, Clerk can contribute to the CLS score. You can read more about CLS here.
Follow this guideline only in the case your CLS score is higher than 0.2 and Clerk elements are above the fold.
In order to prevent content from shifting, there is a need of reserving a placeholder for Clerk recommendations before Clerk.js inject them. To do so, we need to add a minimum height based on expected content height.
Example of code:
.clerk-slider-wrapper {
min-height: 300px; /* Adjust based on expected content height */
width: 100%;
}
Making API calls #
You can use Clerk.js to make API calls, by using the built in function Clerk("call")
.
This function takes 3 arguments:
- An API endpoint
- A JSON dictionary of parameters
- A callback function to handle the response
Requests #
Below is an example script that request the 10 most popular products and logs the response to the console. The response contains a JavaScript object with the status of the API call, and the result.
Script #
function popularProducts(){
Clerk("call",
"recommendations/popular",
{
limit: 10,
labels:["Home Page / Bestsellers"]
},
function(response){
console.log(response);
},
function(response){
console.error(response);
}
);
}
Response #
__clerk_cb_1(
{
"status":"ok",
"count":72,
"facets":null,
"result":[399,410,551,338,403,439,425,402,406,456]
}
);
Callbacks #
When you make API calls, you can use callback functions to handle the response as you see fit. The functions take response
as an argument which contains all data returned by the API.
Below is an example that creates a list of HTML elements linking to categories matching the query “men”.
Clerk("call",
"search/categories",
{
'query': "men",
'limit': "10"
},
function(response) {
var cat = response.categories;
if (cat.length > 0) {
let heading = document.createElement('h3');
heading.textContent = 'Related Categories';
document.querySelector('#your-target').append(heading);
}
for (var index in cat) {
var clerkName = cat[index].name;
var clerkUrl = cat[index].url;
let link = document.createElement('a');
link.href = clerkUrl;
link.textContent = clerkName;
document.querySelector('#your-target').append(link);
}
}
)
Add-To-Cart Buttons #
These buttons work differently for each platform and the functionality can change depending on plugins you use. Because Clerks designs consist of HTML & CSS, you can usually add this functionality if you understand how it works on your site.
General Approach #
Some add-to-cart buttons require Javascript to run, in order for them to work. In these cases, you can to add the functionality to Clerks existing cart
method. Check how to do this in our
developer docs here.
Inspect the add-to-cart button to identify the code associated with it, e.g. on a category page. The code will usually be a <div>
or a button
element. Copy the whole button HTML.
Copy and paste this code into your Design. Now you need to identify the variables in the code. Most often, you need to find where the code uses:
- Product ID
- Product quantity
Replace the values for the product ID with the Liquid variables for these data points. The ID will always be {{ product.id }}
and the quantity will differe depending on how you are sending the data. For this example it could be {{ product.qty }}
.
Paste your code into the HTML of your Design and test it to make sure it works.
Example #
The add-to-cart button below is a <div>
that has the class button-container
:
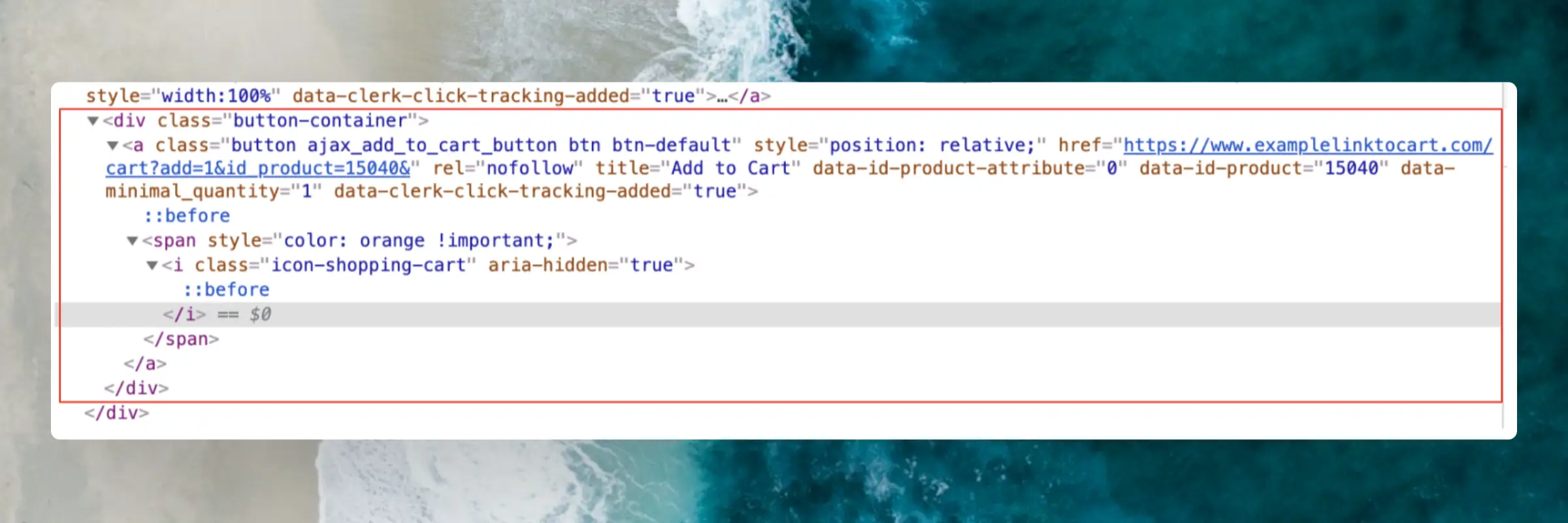
The quantity is found inside the cart link after /cart?add=
and the Product ID is found just after &id_product=
.
The product ID is also referenced in data-id-product
, and the product quantity is referenced in .data-minimal_quantity
These values should be replaced with Liquid tags in the Design so the appropriate product IDs and quantities are used in the HTML output.
With these changes, the final add-to-cart button will look like this:
<div class="button-container">
<a
class="button ajax_add_to_cart_button btn btn-default"
style="position: relative;"
href="https://www.examplelinktocart.com/cart?add={{ product.qty }}&id_product={{ product.id }}&"
rel="nofollow"
title="Add to Cart"
data-id-product-attribute="0"
data-id-product="{{ product.id }}"
data-minimal_quantity="{{ product.qty }}">
<span style="color: orange !important">
<i class="icon-shopping-cart" aria-hidden="true"></i>
</span>
</a>
</div>
Common console errors #
Clerk.js logs many errors to the console, which you can use to debug issues.

By clicking the error link you will get more information about what went wrong, which you can use to debug the error yourself, or to send to our support team who will help you out. Below you will find a list of the most common errors.
LogicalError: Unknown content #
This error will show if the snippet you inserted is referencing a
Content that does not exist, in the data-template
attribute.
To fix it, make sure that the name in the embedcode matches a Content block you have created in my.clerk.io.
You can click Edit Content for any Content, to see what the reference should be.

AuthenticationError: Invalid API endpoint #
This error happens if you have used the class clerk
in your HTML somewhere. This class is reserved for use with our snippets, as Clerk.js uses this class to identify the elements to render.
To fix it, make sure that you name the class something else, like clerk-product
.
ParsingError: Invalid type of argument product #
This error means that the supplied ID for a product has a wrong type or syntax.
For example, if your product-ID’s are integers they also need to be so in the embedcode. Also, remember the brackets around the ID, to make it a list.
<span
class="clerk"
data-template="@product-page"
data-products="[123]">
</span>
ParsingError: Invalid type of argument category #
This error means that the supplied ID for a category has a wrong type or syntax.
In most cases, it happens if the placeholder in the category embedcode has not been replaced by an actual ID:
<span
class="clerk"
data-template="@category-page"
data-category="INSERT_CATEGORY_ID">
</span>
The output of the code should contain the ID of the category:
<span
class="clerk"
data-template="@category-page"
data-category="257">
</span>
If you copied the snippet manually, make sure to select your shop system in the Choose your platform dropdown before copying the snippet. It will then change to include your platforms logic to select the correct category ID.
If your platform is not listed, you need to manually add the logic to select the correct category ID based on your webshops functionality.
AuthenticationError: Incorrect public API key #
This error will show if the public API key you have provided, does not match any account in Clerk.io.
To fix this, login to my.clerk.io, and go to Settings > API Keys. Here you can find the Public API Key which you can then add to your Clerk.js tracking script either directly in the code, or in the settings for your integration, depending on your platform.
Sending sales data from a POS/ERP system #
For some webshops, it’s relevant to upload sales-data from other systems than the actual webshop, for example if you want to optimise the algorithm based on sales from a physical store, or B2B store.
Clerk.io does not differentiate between orders from various sources - as long as you can provide an ID, a timestamp and a list of products that were bought, they can be uploaded to Clerk.io.
The recommended approach is to use the CRUD API as that allows you to automate the task entirely. By implementing these API calls, you can send order data directly to your Store in Clerk.io.
Simply create a POST call to the /orders endpoint in your ERP system or webshop, run the job at regular intervals, e.g. once a month, and you will be able to use offline orders to boost your online recommendations and search results.
Alternatively, you can upload a CSV file manually, without the need to code anything. You can read more about CSV files here.
Currency conversion #
There are multiple ways of working with currency conversion in Clerk.io. A simple way to make it work is outlined below.
Send Price Objects #
Clerk needs to know the prices of each product in the different currencies. The easiest way to do this is to send them as a string-encoded JSON object of formatted prices, with the currency ISO as their key, in your Data Feed.
"products": [
{
"id": 1,
"name": "Cheese",
"description": "A nice piece of cheese.",
"price": 100,
"list_price": 50,
"categories": [25, 42],
"image": "http://example.com/images/products/1.jpg",
"url": "http://example.com/product/1",
"prices_formatted": "{'USD':'$100', 'EUR':'€ 87.70', 'GBP':'£ 68.68'}"
},
{
"id": 2,
"name": "A pound of nuts",
"description": "That's a lot of nuts!",
"price": 150,
"categories": [1],
"image": "http://example.com/images/products/2.jpg",
"url": "http://example.com/product/2",
"prices_formatted": "{\"USD\":\"$150\", \"EUR\":\"€142\", \"GBP\":\"£120\"}"
}
]
Create a Formatter #
In Clerk.js you can define JavaScript functions, that can be used with your Designs.
Here you can define a function that takes your price-dict as argument, and returns the price for a specific currency, based on frontend logic of your choice.
Make sure to replace currency
with the currently chosen currency from the frontend.
Clerk('config', {
key: 'Your_API_Key',
formatters: {
currency_selector: function (price_list) {
const currency = "EUR";
price_groups_obj = JSON.parse(price_list)
return price_groups_obj[currency];
}
}
});
Use Formatter #
After defining the formatter, it can be used in your design with the below syntax:
<div class="price">
<span class="price">
{{ product.prices_formatted | currency_selector }}
</span>
</div>
Customer-specific prices #
To display completely unique prices based on which customer is logged in, create an Event in Clerk.io that inserts the correct price before the products are rendered.
Events are Javascript functions that are run before or after Clerk.io shows products.
This method is possible to use if you can look up prices from your server, directly from a Javascript function, in the frontend based on a product ID and a customer ID.
For showing individual customer prices, the code should run right after the response.
Below is an example of a simple event.
<span class="clerk" data-template="@home-page-popular"></span>
<script>
Clerk('on', 'response', function(content, data) {
console.log(data.result);
});
</script>
The function takes the argument data which is the entire response that Clerk.io sends back from the API.
Individual Customer Prices Example #
If you need to display completely unique prices based on which customer is logged in, you need to setup an Event that inserts the correct price after the products are rendered.
Events are Javascript functions that are run before or after Clerk.io shows products.
This approach assumes that you can can look up prices from your server with an AJAX call in the frontend based on e.g. a product ID and a customer ID.
The best way is to first create a placeholder price container in your design, and then replace it with the price returned from your AJAX call.
An example:
<div class="clerk-price-container">
<span class="clerk-price">
Loading price...
</span>
</div>
You can then use the Clerk event to wait for the products to be rendered, make a request to your price server using the product ID and the ID of the customer, before replacing it in the HTML.
Here is an example of doing this:
<script>
var customer_id = INSERT_CUSTOMER_ID;
Clerk("on", "rendered", function(content, data){
for (i = 0; i < data.product_data.length; i++) {
var product = data.product_data[i];
var custom_price = FETCH_PRICE_FROM_SERVER(product.id,customer_id);
let price_container = document.querySelector(`[data-clerk-product-id='${product.id}'] .clerk-price`);
price_container.innerText = custom_price;
}
})
</script>
The above code assmues that you can identify a logged in customer with INSERT_CUSTOMER_ID
and that you have a function like FETCH_PRICE_FROM_SERVER
that returns the price for the product based on the customer.
price_container
is used to target the right product based on the ID that’s available in data-clerk-product-id
, which is appended to all products by Clerk.js.
Finally it replaces the inner text, “Loading price…” in this example, with the price returned from your AJAX call.
Customer Group Prices #
The setup of customer group prices consists of 4 steps:
- Include the various prices in the data feed
- Include a variable fetching the current customer group ID
- Create a function to fetch the relevant price
- Show the price in the Design
Include Price Objects #
Start by adding an attribute to all products which contains all of the various pricing options, making sure to correlate each price to a particular customer group.
This should be sent as a string-encoded JSON object. For example:
"customer_group_prices": "{\"GROUP1\":100,\"GROUP2\":202,\"GROUP3\":309}"
Customer ID Variable #
Add a dynamic global variable to Clerk.js which fetches the customer-group ID of the current customer and adds it as the value.
The Customer Group ID value must be equivalent to the key of its corresponding price in the Data Feed. For example, a user that should see the price for group 2, then the ID should be “GROUP2”.
Clerk('config', {
globals: {
customer_group: "GROUP2"
}
});
Fetch Price #
You can now create a Formatter, which takes the customer_group as an argument and returns the relevant price.
Do this by writing a function that fetches the price from the specific customer group as the key in the price-dict based on the customer_group ID.
Add this in the Clerk.js config. Below is an example called getPrice
:
Clerk('config', {
globals: {
customer_group: "GROUP2"
},
formatters: {
getPrice: function (product) {
const gid = window.Clerk._config.globals.customer_group;
if (product.customer_group_prices) {
const map = JSON.parse(product.customer_group_prices);
if (map[gid]) {
return map[gid];
} else {
return product.price;
}
} else {
return product.price;
}
}
}
});
Show Price #
When the final_price formatter has been created, you can name it directly in your Design, along with the customer_group_prices list that you created in step 1:
<li style="text-align: center; width: 180px;">
<a href="{{ product.url }}">
<img sre="{{ product.image }}" />
{{ product.name }}
</a>
<div class="price">
{{ product | getPrice }}
</div>
</li>
HTTP Auth Syncing #
Often HTTP authentication is used on staging sites to avoid unwanted visitors.
This will, in many cases block the Clerk importer as well and typically display a 401 Unauthorized error in the sync log.
You can easily verify the importer by inserting the authentication info in the import URL like below, in Data Sync at my.clerk.io:
http://USER:PASS@www.ewoksRus.com/JSONFEED
No Tracked Orders #
Clerk.io needs to continuously track sales from the webshop to keep results up-to-date with your customers’ behaviour. However, some settings in a webshop may cause the sales-tracking to fail.
Below, you can find out how to debug the sales-tracking when using a Clerk.js setup and see what the most common issues and solutions are.
Before you start #
Make sure that you have installed:
- The Clerk.js tracking script on all pages
- The Sales-tracking script on your Order Success page.
These are required to track sales in general when you use a Clerk.js setup.
Check Logs #
In most cases, sales tracking fails due to errors in the visitor IDs or product IDs of the sale call that is send to Clerk after a purchase has been completed. To debug this, you’ll have to make a test order.
However, in some cases it might be related to the sales tracking script itself and can debugged by looking at the logs in my.clerk.io > Data > Logs.
If the sales-tracking is failing because of an error in your script, you will often be able to see the error in this page. Click Details to see more.

If you cannot see any errors in logs, the easiest way to identify other sales-tracking issues is to place a test order.
Test Order Debugging #
In this example, we use Chrome to show how to debug sales tracking with a test order, but other browsers have similar features.
- On your webshop, put a couple of products in the basket.
- Proceed to Checkout.
- Before placing the order, open your browsers Console.
- Find Network, and search for " clerk".
- Place the order, so you see the order confirmation page.
- Click the call that starts with sale (normally sale?key=…).
Here you will see the data that is sent to and received by the sales-tracking API endpoint. Click Preview to identify any errors that can cause sales to not be tracked.
Below are common errors associated with sales-tracking.
Invalid Syntax In Argument: products #
This error happens if the product IDs you send have a wrong syntax. The most common errors are:
- The product-IDs are string-encoded in the sales-tracking, but you are using Integers in Clerk.io or vice-versa.
- The list of product IDs contain text-formatting characters instead of pure JSON:
"products":\[\\"id"\\:\\"123-m"\\\]
.
Missing Argument X #
This means that you are not sending all the data Clerk.io needs to track the sale.
Make sure you include all the necessary data attributes in the sales-tracking.
No Call Made #
If you can’t see the call to sale even with both scripts installed, then something has caused the Clerk.js script to be loaded incorrectly. Test this by following these steps:
- Open the Console in your browser
- Type in “Clerk”.
- If Clerk.js has not been loaded correctly, you will see a ReferenceError:
Uncaught ReferenceError: Clerk is not defined
If this is the case, you need to check your Clerk.js setup:
- Make sure Clerk.js is installed on all pages.
- Make sure it’s not blocked by other Javascript functionality.
No Clerk Impact #
If you successfully track sales in my.clerk.io, but none of them shown as impacted by Clerk.io, you might have an error in your visitor-tracking / click-tracking setup.
Start by making sure that visitor-tracking works, by doing the following:
- Click on any product through Clerk.io’s Search or Recommendations
- Proceed to place an order containing this product
- Login to my.clerk.io and go to Order Details
- Wait for the Order to show up.
If visitor-tracking is working, you will see the value and impact from the product you added through Clerk.io, in Tracked Orders:

If you see no value added in the order you placed, the following sections show common errors that could cause this.
API Setups #
If you set up Clerk.io with a custom integration directly with the API, you need to actively enable visitor-tracking. Read how to do in this API article.
Wrong Product IDs #
For visitor-tracking to work, the click- and sales-tracking must track the same product ID’s as the ones we receive in the importer. Usually if this doesn’t work its because you are tracking variant ID’s instead of parent ID’s or the SKU instead of the ID.

To check if this is the issue do the following:
- In my.clerk.io, go to Data > Orders and click the ID of an order you placed.
- If Clerk.io cannot identify the product, you will see an ID and image placeholder:
- Go to Data > Products and search for the name of the product you added. Here you will be able to see the expected ID for the product.
Use this to configure your sales-tracking to use the correct product IDs.
Visitor ID Changes #
Clerk.io uses a visitor ID to identify each individual session, including the products they click and buy. Due to this, the ID’s should stay the same throughout at least the entire session, and preferrably across multiple ones as well.
This visitor ID is created automatically when using Clerk.js to do the setup, but if you use an API setup, or customise your visitor ID’s, you might acciendetally change it.
This error is rare, but you can check the visitor ID by following these steps:
- Open up your Network settings in the browser, and narrow down results to “clerk”.
- Start by checking any of the
undefined
calls which are related to search or recommendations. - In
payload
you can check the current Visitor ID. You will be able to do this for all calls associated with Clerk.io - Proceed to click the product, and place an order with this product.
- On the Order Success page, check your Network again, and find the call to
sale?
. - Make sure that
visitor
in thepayload
, matches the Visitor ID you saw in step 3.
If the visitor
ID’s mismatch, you need to find out why they change. A common cause for visitor ID’s changing could be if you regenerate the ID’s for each new page that is loaded. Update your code to use the same visitor ID for each page.
Upgrade to Clerk.js 2 #
Clerk.js 2 is a faster and much more flexible version of our JavaScript library that makes installing Clerk.io on any webshop a breeze.
However, since the two versions work slightly differently, you need to follow these steps to successfully upgrade.
The two main differences in Clerk.js 2 is:
The Designs in my.clerk.io use the Liquid, but can also easily be created using the Design Editor.
The script must be inserted just before the tag in your webshops template.
Create Designs #
Since Clerk.js 2 has a different approach Designs, you need to create new ones.
You can create your Clerk.js 2 Designs either by redoing them in the Design Editor, or by converting your old code Designs to Liquid, which the below guide explains how to do. Below is a description for how to convert your old code Designs to Liquid.
Option 1: Design Editor Designs #
- Go to my.clerk.io > Recommendations/Search > Designs > New Design.
- Select the a design type other than Blank and give it a name. We recommend adding “V2” so it’s obvious that you are using Clerk.js 2 designs for this.
- In the Design Editor, click any of the existing elements like the name, image, button, etc. to edit it, or add new elements to the Design.
- Click Publish Design when you are done, and go to Step 2 in the guide.
- Lastly, go to Recommendations/Search > Content and change your Clerk.io Content to use your new Design, then click Update Content.
- This will temporarily cause them to not show up on your webshop, until you have inserted Clerk.js 2 as decribed further down in this guide.
Option 2: Converting Designs #
Since Clerk.js 2 uses the more flexible template language Liquid, you need to convert the Designs into this language.
- Start by going to my.clerk.io >Recommendations/Search > Designs > New Design.
- Select Blank > Code and give it a name. We recommend adding “V2” so it’s obvious that you are using Clerk.js 2 designs for this.
- Click Create Design.
- This will give you a blank design with Product HTML and CSS that you can use.
- Go back to the design overview and click Edit Design for your Clerk.js 1 Design. We recommend doing this in a new tab so you can easily copy the code.
- Now you need to copy over the old Clerk.js 1 Design to your new Clerk.js 2 Design.
- You will notice that there is no Container Code in your new one.
- This is because Liquid uses for loops to render all the products.
- Copy your old Product HTML inside the for-loop, your old Container Code around it and copy the CSS as well.
- Convert the Design into Liquid’s syntax. The main difference is that the old Designs used the syntax
{{ formatter attribute }}
while v2’s syntax is{{ product.attribute | formatter }}
. - Go through all of your attributes and change them to the new format.
- If you are using
{{#if}}
or{{#is}}
statements, these need to be converted as well. Use the syntax{% if product.attribute %}
{% else %}
{% endif %}
. - Delete
id="{{ $id }}"
and the class:target
from the container code in the Clerk.js 2 version as they are no longer supported. - Below is an example of a Clerk.js 1 design, and the fully converted version:
Clerk.js 1 Design #
// Product HTML
<li class="clerk-product">
<a href="{{ url }}">
<img src="{{ image }}" />
<div class="clerk-product-name">{{ name }}</div>
<div class="clerk-price-wrapper">
{{#if list_price}}
<div class="clerk-old-price">
<s>Price {{ money_eu list_price }}</s>
</div>
<span class="clerk-new-price">Price {{ money_eu price }}</span>
{{else}}
<div class="clerk-product-price">Price {{ money_eu price }}</div>
{{/if}}
</div>
</a>
<div class="clerk-cta-button btn button">Buy Now</div>
</li>
// Container Code
<h2>{{ headline }}</h2>
<ul id="{{ $id }}" class=":target clerk-slider"></ul>
<!-- This code creates the slider by its ID. -->
<script type="text/javascript">
Clerk.ui.slider("{{ id }}").init();
</script>
Clerk.js 2 Design #
<h2>{{ headline }}</h2>
<ul class="clerk-slider">
{% for product in products %}
<li class="clerk-product">
<a href="{{ product.url }}">
<img src="{{ product.image }}" />
<div class="clerk-product-name">{{ product.name }}</div>
<div class="clerk-price-wrapper">
{% if product.list_price %}
<span class="clerk-old-price"><s>Price {{ product.list_price | money_eu }}</s></span>
<span class="clerk-new-price">Price {{ product.price | money_eu }}</span>
{% else %}
<div class="clerk-product-price">Price {{ product.price | money_eu }}</div>
{% endif %}
</div>
<div class="clerk-cta-button btn button">Buy Now</div>
</a>
</li>
{% endfor %}
</ul>
- Now click Update Design to save the changes.
- Lastly, go to Recommendations/Search > Content and change your Content block to use your new Design.
- Click Update Content. This will temporarily cause them to not show up on your webshop, until you are done with Step 2. Choose the new Design for all Content that should be updated.
Replace the script #
- Start by locating the template file that is used to show all pages of the webshop, and where the original Clerk.js script is found near the bottom.
- Remove the old Clerk.js script from the file. It will look something like this:
<!-- Start of Clerk.io E-commerce Personalisation tool - www.clerk.io -->
<script type="text/javascript">
window.clerkAsyncInit = function() {
Clerk.config({
key: 'public_api_key'
});
};
(function(){
var e = document.createElement('script'); e.type='text/javascript'; e.async = true;
e.src = document.location.protocol + '//api.clerk.io/static/clerk.js';
var s = document.getElementsByTagName('script')[0]; s.parentNode.insertBefore(e, s);
})();
</script>
<!-- End of Clerk.io E-commerce Personalisation tool - www.clerk.io -->
- Go to my.clerk.io > Settings > Tracking Code. This contains the Clerk.js 2 code.
- Copy this code, insert it just before the
</head>
tag in the template, then save it.
Congratulations! You are now running on the much-improved Clerk.js 2 setup! You can see the full documentation for Clerk.js 2 here.
Handling require.js #
This section only applies when using Clerk.js 1.
In some setups, Require.js stops Clerk.js from loading, which means that no sliders or search results will be shown. When this happens, the following error will be shown in your console:
Uncaught ReferenceError: Clerk is not defined
There are two ways to handle Require.js. Both approaches requires you to make changes to the tracking-script, that you have inserted in the bottom of all pages.
Include “clerk” in Require.js #
The best approach is trying to get Require.js to recognize Clerk.io.
You can do this by inserting require(\['clerk'\], function() {});
in the bottom of the tracking script:

Ignoring Require.js #
If the above solution doesn’t work, its possible to ignore Require.js.
You can do this by inserting window.\_\_clerk\_ignore\_requirejs = true;
in the top of the tracking script:

After using one of these approaches, Require.js will now be compatible with Clerk.io.