Syncing data #
Clerk syncs each webshop domain, called a Store, as its own unique instance, which is accessed by a set of API Keys, found in the Clerk admin:
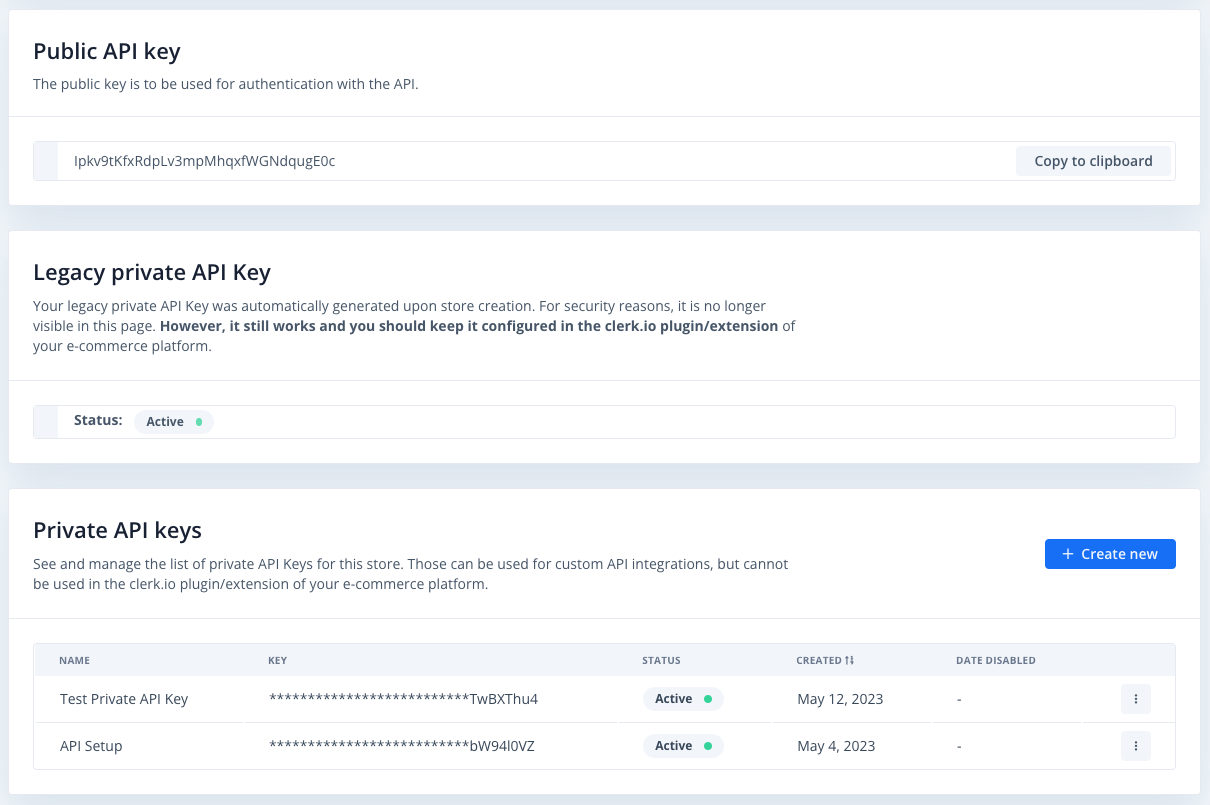
These include a Public key, which gives access to endpoints exposing non-sensitive data, and a Private Key. The Private Key, when combined with the Public key, allows you to work with data on the Store and access sensitive data, such as customer and order information.
CRUD API #
You can sync your data using the CRUD API endpoints, which allow you to get, post, update, and delete resources on demand.
curl --request POST \
--url 'https://api.clerk.io/v2/products' \
--header 'accept: application/json' \
--header 'content-type: application/json'
-d '{"key": "Ipkv9tKfxRdpLv3mpMhqxfWGNdqugE0c",
"private_key": "osLqDAs5s2tlf3adpLv6Mp1hxxf6GfdDuSE0c2ftT2ot5F3",
"products":[
{
"id": 123,
"name": "Green Lightsaber",
"description": "Antiuque rebel lightsaber.",
"price": 99995.95,
"brand": "Je’daii",
"categories": [987, 654],
"created_at": 1199145600
},
{
"id": 789,
"name": "Death Star Deluxe",
"description": "Death Star. Guaranteed idiot proof. "
"price": 99999999999999.95,
"brand": "Imperial Inc.",
"categories": [345678],
"created_at": 11991864600
}
]
}'
The available ressources are:
One of Clerk’s primary differentiators is that there’s no learning period, since we can utilize all existing orders from day one to understand current customer behavior.
Data feed #
In addition to using the CRUD API, it’s highly recommended to add a backup sync method.
After all, many things can go wrong with API calls. For example, your price server might crash, or a product attribute might contain an error that causes several calls to fail. To avoid these issues, consider using a Data Feed as a daily backup for your CRUD calls.
The feed is a JSON file containing the current state of a webshop’s catalog. Any data available in the feed when it is loaded will be what Clerk works with, except for orders, which are logged and do not need to be included in the feed after the first import. Using the data feed is also a great way to preload Clerk with orders.
{
"products": [ ... ],
"categories": [ ... ],
"orders": [ ... ],
"customers": [ ... ],
"pages": [ ... ],
"config": {
"created": 1567069830,
"strict": false
}
}
The data feed should be available at a URL that can be accessed by the importer, which you configure in the my.clerk.io backend:
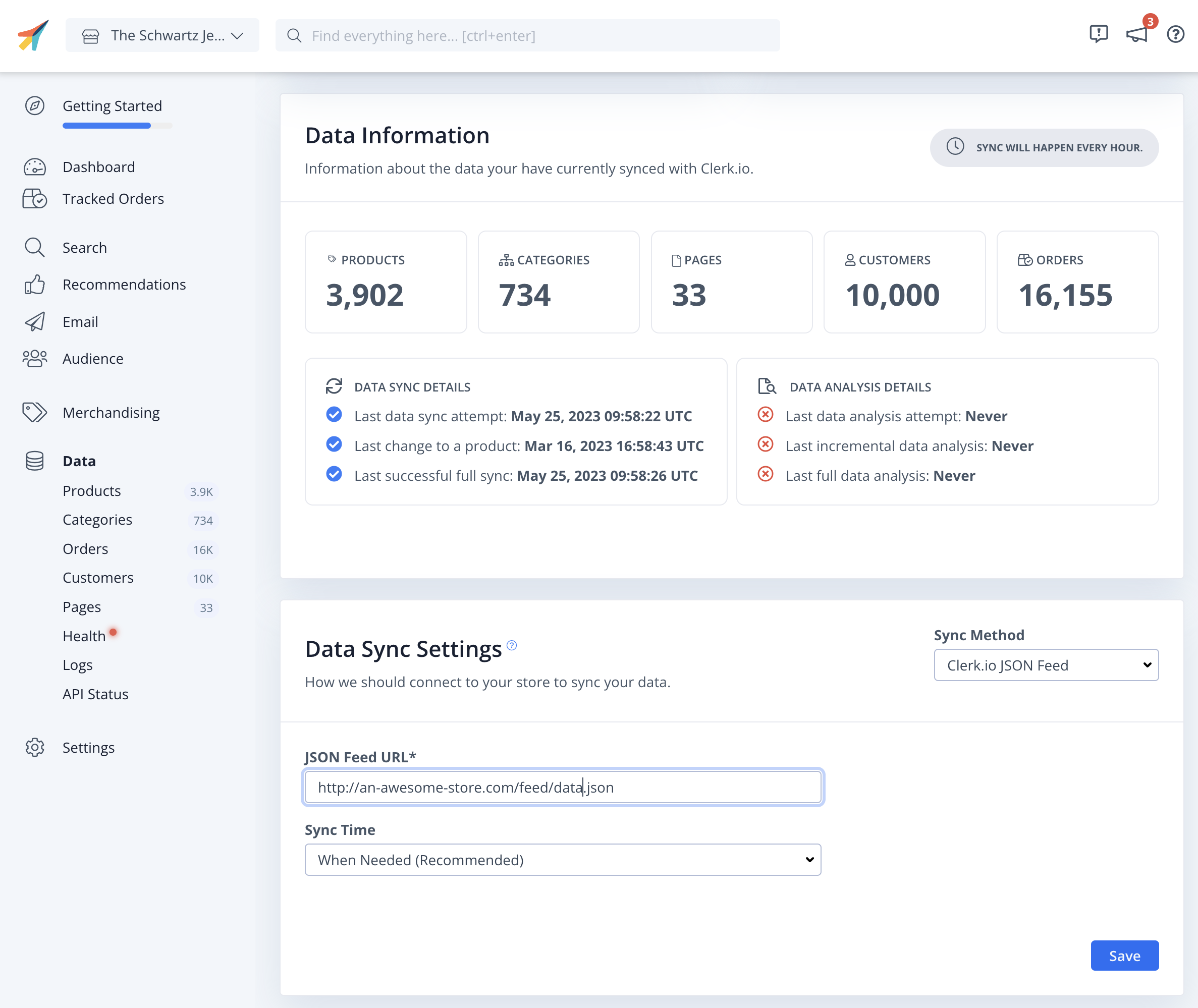
You can secure the feed, so only our importer can access it.
Retrieving data #
Once data is synced, the AI analyzes it and builds intelligent indexes that can be retrieved through unique endpoints depending on the use-case.
For example, to fetch the hottest products, you can use the recommendations/trending endpoint, and to display the top products for a search on “star wars,” you can use the search/predictive endpoint.
Endpoints #
All endpoints require you to send the public API key.
Endpoints that return results also require the " limit" argument to control the number of results to be returned.
Additional arguments depend on the endpoint you are calling. For example, complementary products require a list of product IDs to find accessories for, and any search-related calls need the “query” parameter to find matches, and so on.
You can find the necessary arguments for all endpoints in our API documentation.
By default, Clerks API returns all available results, but if necessary, Filters can be used to define a subset of matches.
Recommendations Example #
curl --request POST \
--url 'https://api.clerk.io/v2/recommendations/trending' \
--header 'accept: application/json' \
--header 'content-type: application/json'
-d '{"key": "Ipkv9tKfxRdpLv3mpMhqxfWGNdqugE0c",
"limit": 30,
"labels": ["Homepage - Trending"]
}'
Search Example #
curl --request POST \
--url 'https://api.clerk.io/v2/search/predictive' \
--header 'accept: application/json' \
--header 'content-type: application/json'
-d '{"key": "Ipkv9tKfxRdpLv3mpMhqxfWGNdqugE0c",
"limit": 30,
"query": "star wars",
"labels": ["Search - Predictive"]
}'
Visualising results #
Clerk’s API always returns the IDs of the matches it found when returning results.
// Call
curl --request POST \
--url 'https://api.clerk.io/v2/recommendations/trending' \
--header 'accept: application/json' \
--header 'content-type: application/json'
-d '{"key": "Ipkv9tKfxRdpLv3mpMhqxfWGNdqugE0c",
"limit": 5,
"labels": ["Homepage - Trending"]
}'
// Response
{
"status": "ok",
"result": [
12793,
13827,
12693,
12791,
1546
],
"count": 3902,
"facets": null
}
To visualize your data, you can make API calls serverside, retrieve the IDs of the matching products, and then fetch all the product-specific information from your webshop platform or PIM before rendering them.
Clerk’s API can also be configured to send back any resource-specific information that you send to Clerk, such as prices, brand names, category URLs, blog cover images, and more. With this, you often don’t need to make individual calls to your PIM before showing results, which will load your page faster.
// Call
curl --request POST \
--url 'https://api.clerk.io/v2/recommendations/trending' \
--header 'accept: application/json' \
--header 'content-type: application/json'
-d '{"key": "Ipkv9tKfxRdpLv3mpMhqxfWGNdqugE0c",
"limit": 30,
"labels": ["Homepage - Trending"],
"attributes": ["id", "name", "price", "image", "url"]
}'
// Response
{
"status": "ok",
"result": [
12793,
13827,
12693,
12791,
1546
],
"count": 3902,
"facets": null,
"product_data": [
{
"id": 12793,
"image": "https://admin.davidshuttle.com/media/catalog/product/cache/2aecdb890d2a6ac64962b1f6d4fcec89/2/8/2807199-baccarat-eye-small-oval-red-vase.jpg",
"name": "Baccarat Eye Small Oval Red Vase",
"price": 520.0,
"url": "/"
},
{
"id": 13827,
"image": "https://admin.davidshuttle.com/media/catalog/product/cache/2aecdb890d2a6ac64962b1f6d4fcec89/1/t/1transatvj.jpg",
"name": "Sabre Transat Garden Green 22cm Soup Spoon",
"price": 13.96,
"url": "/"
},
{
"id": 12693,
"image": "https://admin.davidshuttle.com/media/catalog/product/cache/2aecdb890d2a6ac64962b1f6d4fcec89/2/t/2transatdl.jpg",
"name": "Sabre Transat Light Blue 22cm Dinner Fork",
"price": 13.96,
"url": "/"
},
{
"id": 12791,
"image": "https://admin.davidshuttle.com/media/catalog/product/cache/2aecdb890d2a6ac64962b1f6d4fcec89/1/8/1845244-baccarat-dom-perignon-champagne-flute-_set-of-2_.jpg",
"name": "Baccarat Dom Perignon Champagne Flute (Set of 2)",
"price": 260.0,
"url": "/"
},
{
"id": 1546,
"image": "https://admin.davidshuttle.com/media/catalog/product/cache/2aecdb890d2a6ac64962b1f6d4fcec89/m/a/maison-berger-ocean-breeze-1-litre-lamp-refill.jpg",
"name": "Maison Berger Ocean Breeze 1 Litre Lamp Refill",
"price": 29.0,
"url": "/"
}
]
}
Tracking #
Lastly, tracking must be added to keep Clerk’s AI up-to-date and personalize a visitor’s results during their session. Clerk tracking is setup in 4 steps:
- Generating a session ID for each visitor
- Adding descriptive tracking labels to API calls that return results, which are used for showing analytics for individual endpoints
- Logging a visitor’s clicks on products shown by Clerk
- Logging each order made on the webshop
Visitor (Session) ID #
The session ID is also called the Visitor ID with Clerk. It is strictly required to log a user’s activity during a session on the webshop, including products clicked, searches made, and categories browsed. This activity is stored for each domain, and Clerk never shares it across stores.
For example, you could use PHP’s uniqid(), function to generate IDs that are unique for at least the current session. Once generated, this ID must be included in all calls to Clerk for tracking to work.
<?php
session_start();
$current_visitor = uniqid(); //Example: "646f1f0584371"
$_SESSION["clerk_visitor_id"] = $current_visitor;
?>
Labels #
Labels must be added to any call that returns results, such as search results or alternatives on a product page. The labels argument is a list containing at least one string, which should be the label of this call.
We recommend using labels that contain the page each call is used on and the type of results it shows, like “Homepage - Trending”. This makes them easy to distinguish in analytics.
curl --request POST \
--url 'https://api.clerk.io/v2/recommendations/trending' \
--header 'accept: application/json' \
--header 'content-type: application/json'
-d '{"key": "Ipkv9tKfxRdpLv3mpMhqxfWGNdqugE0c",
"limit": 30,
"labels": ["Homepage - Trending"],
"visitor": $_SESSION["clerk_visitor_id"]
}'
Logging clicks #
Then, you should log each click on a Clerk product with log/click. It’s important to only make this call when the product clicked is actually shown by Clerk and not the webshop platform itself; otherwise, it will look like all products are found through Clerk.
Include the product id product
, and ideally also the api
endpoint and the labels
from the API call that showed this product. If this is not included, Clerk will backtrack the click to the last API call that showed this product.
curl --request POST \
--url 'https://api.clerk.io/v2/recommendations/trending' \
--header 'accept: application/json' \
--header 'content-type: application/json'
-d '{"key": "Ipkv9tKfxRdpLv3mpMhqxfWGNdqugE0c",
"labels": ["Homepage - Trending"],
"api": "recommendations/trending",
"visitor": $_SESSION["clerk_visitor_id"],
"product": 12793
}'
Logging sales #
Lastly, use log/sale to track each order as soon as it is placed on the webshop. With the visitor ID included in this call, Clerk will understand which products were shown, clicked on, and finally purchased. This allows the AI to always stay up-to-date and change results on the fly based on customer behavior.
Important:
- The
id
of products should match the IDs that are logged withlog/click
. E.g. for configurable products you should track the parents ID in bothlog/click
andlog/sale
regardless of the variant bought. price
is the unit price for products. Clerk multiplies it by the quanitity in our analytics.
curl -X POST \
-H 'Content-Type: application/json' \
-d '{"key": "Ipkv9tKfxRdpLv3mpMhqxfWGNdqugE0",
"sale": 567,
"products": [
{
"id": 12793,
"price": 99.95,
"quantity": 2
},
{
"id": 1546,
"price": 14.00,
"quantity": 2
}
],
"customer": 1234,
"email": "theone@matrix.com",
"visitor": $_SESSION["clerk_visitor_id"]}' \
https://api.clerk.io/v2/log/sale
Details of the Visitor ID #
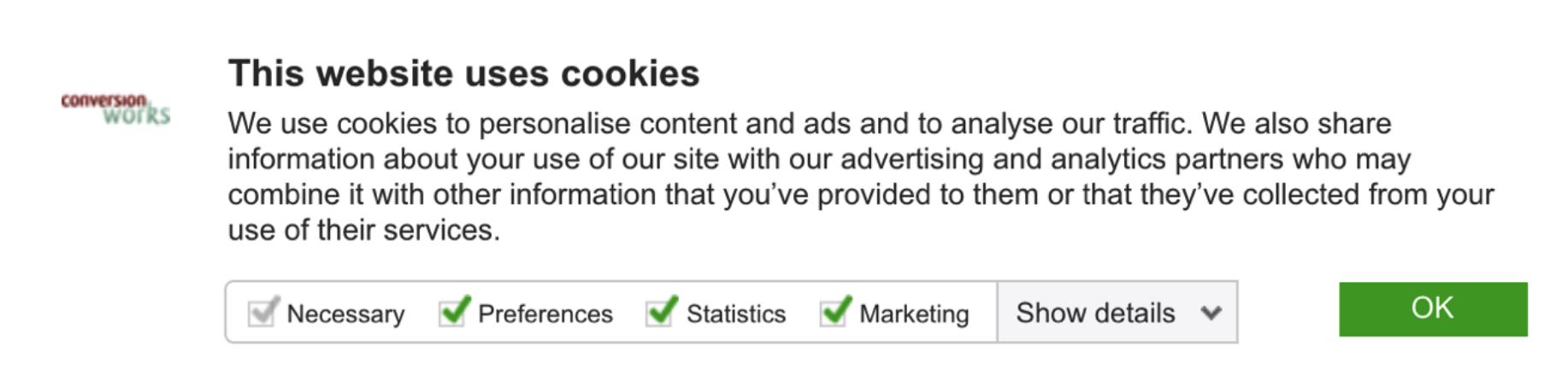
By default, Clerk.io is cookieless and uses anonymous visitor ID’s rather than storing cookies.
The ID is used for providing analytics in Clerk.io’s Dashboards and for on-site functionality like “You Previously Visited” recommendations.
For more details about how Cookieless Personalisation works, check this article.
What do we use the ID for? #
Dashboards, Analytics and Attribution.
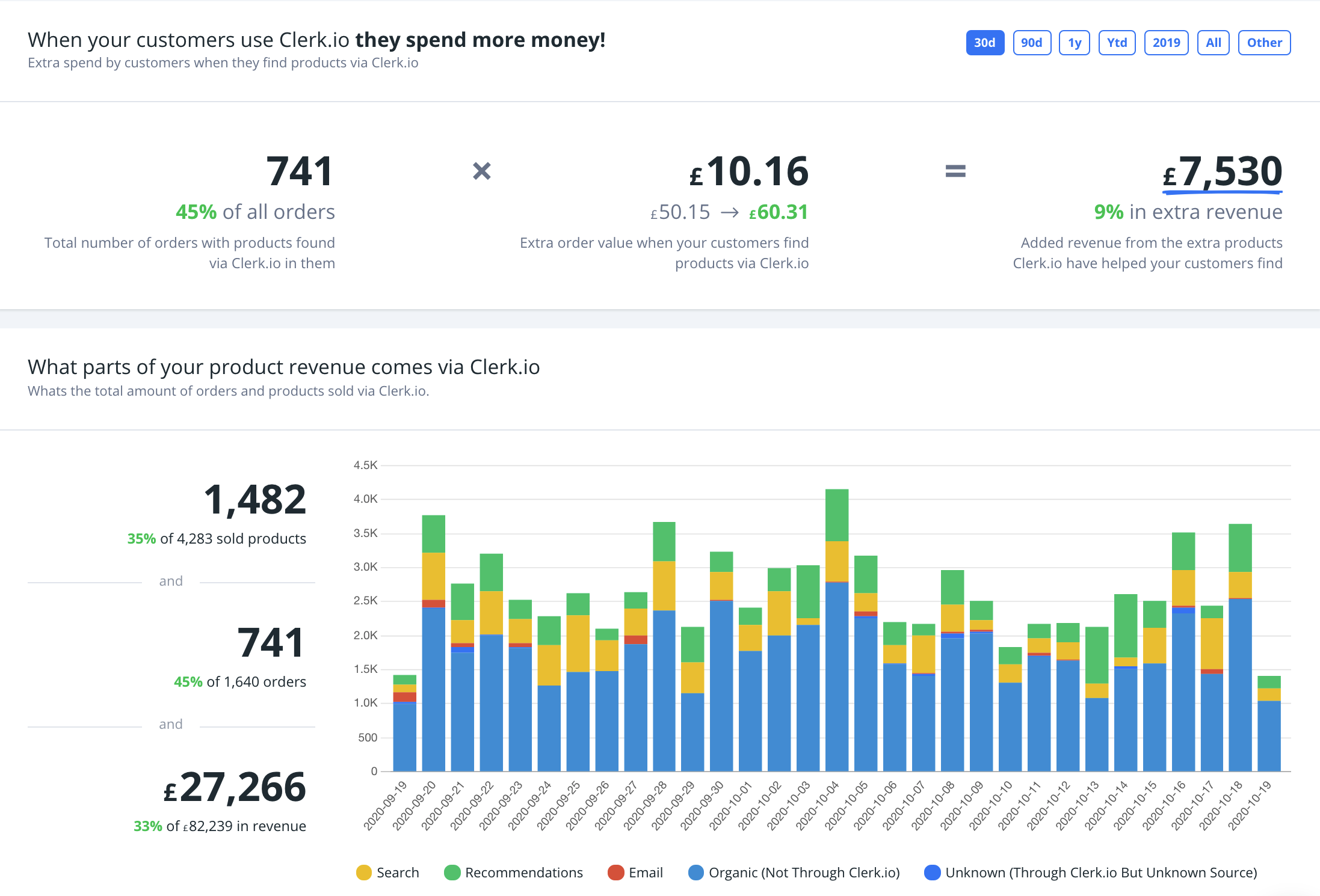
Clerk.io tracks all orders placed on the webshop, and compares the ones impacted by Clerk.io, with the orders not impacted.
Through the visitor ID, we can track when a consumer clicks on a product in a Clerk.io element, and proceeds to place an order containing that product to allow for attribution.
On-site Functionality
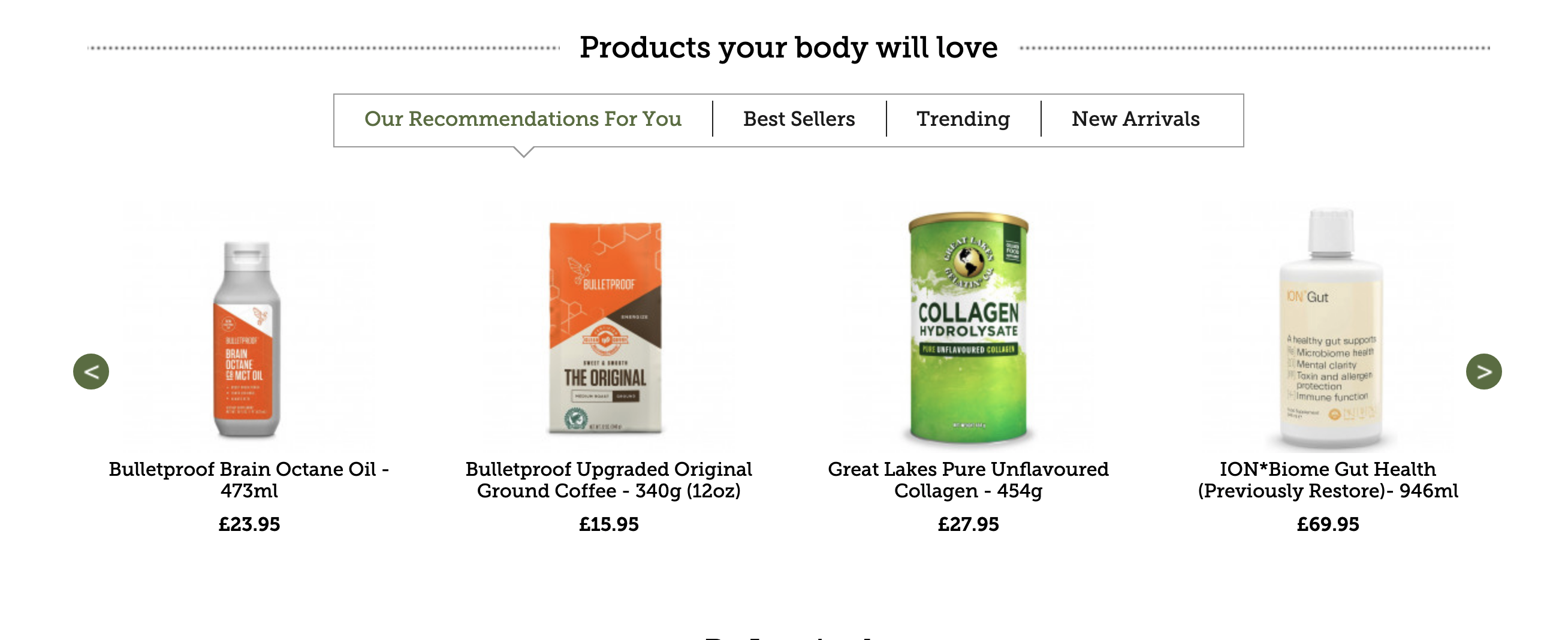
Through the visitor ID, we collect the IDs of products that a consumer browses and the searches they make.
This allows us to personalise recommendations on the webshop, including banners like " Our Recommendations For You" and " You Previously Saw" that show products related to browsing on the webshop.
The visitor ID and how it works #
Every call being made to Clerk.io will contain the anonymous visitor ID described above so it can be used for the above purposes.
When a visitor writes their email address on the webshop and/or places an order, the Visitor ID is attached to their email address if sales-tracking is activated.
An email address in Clerk.io can contain multiple Visitor IDs which can be seen on the customers page in my.clerk.io:
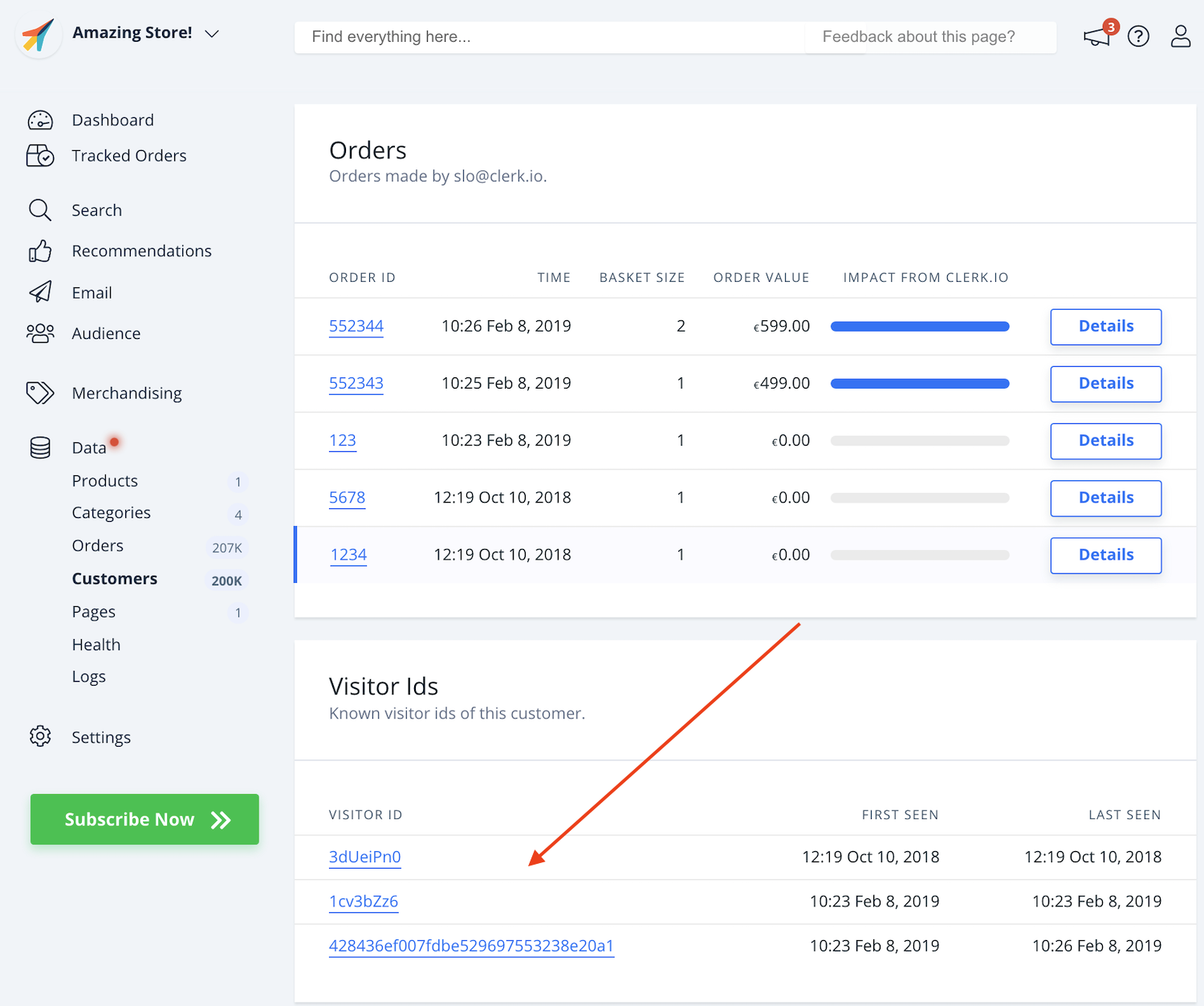
If a user accepts cookies from your site, you can configure Clerk to add a cookie that enables long-term tracking with this generated ID. This is done simply by adding visitor: ‘persistent’ to Clerk.js.
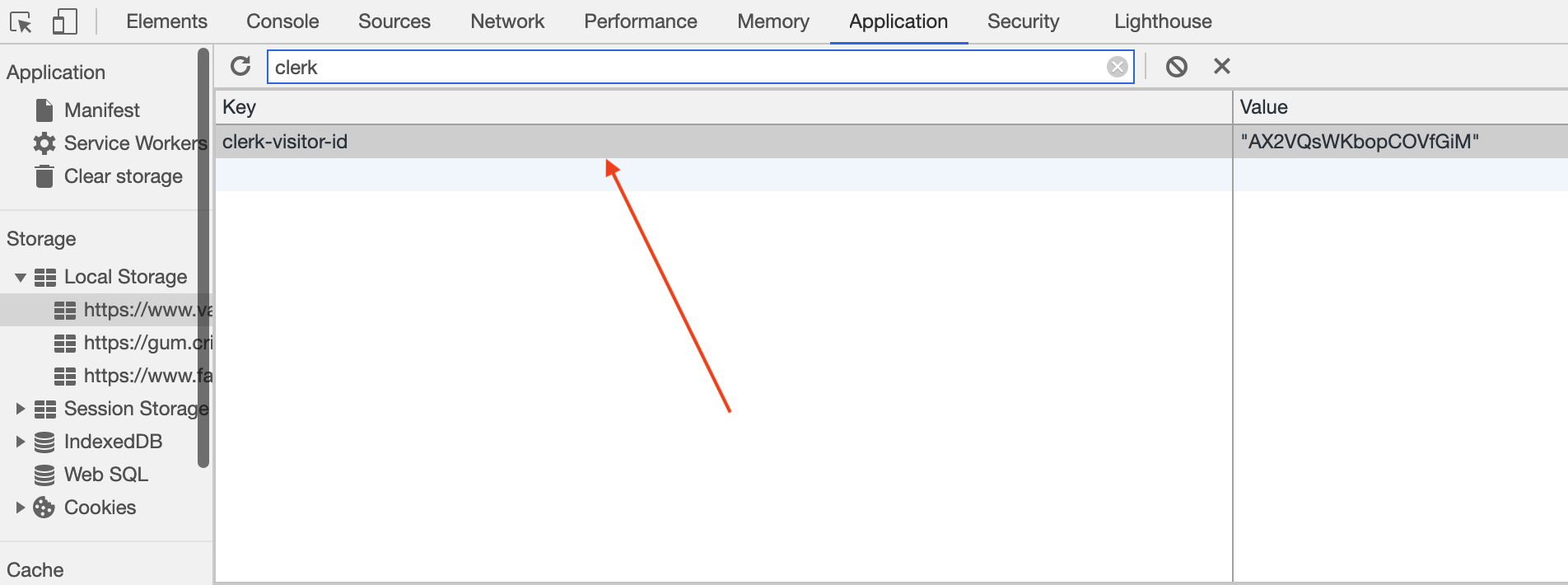
Deactivating the ‘visitor’ ID #
By default, Clerk.js runs in cookieless mode, so if the visitor parameter is not set, we will log the session activity without adding a cookie.
Should you want to allow the visitor to choose not to be tracked in anyway, the ID can be deactivated entirely by adding ‘visitor’: null:
Clerk.js
In setups using Clerk.js, add it to the Clerk.js code for that visitor:
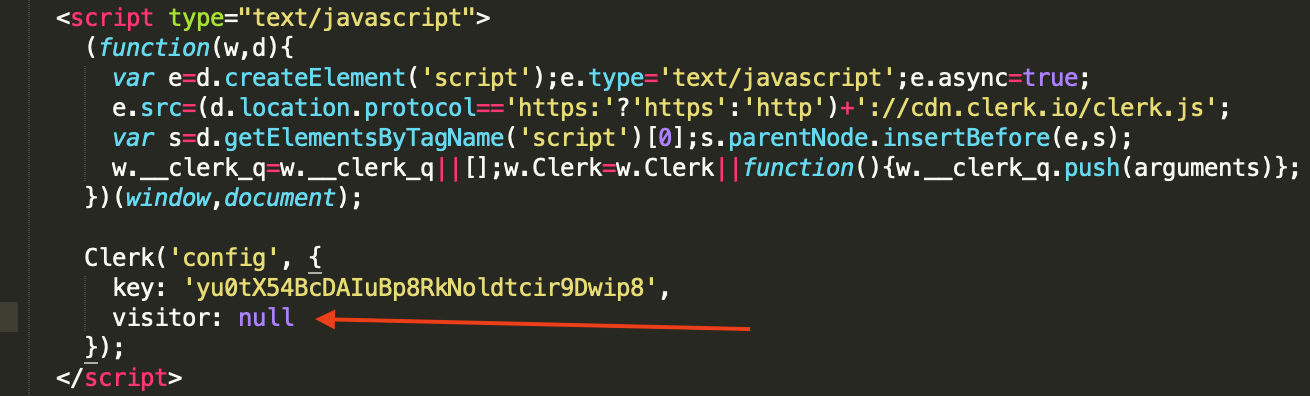
API
If you are using direct API calls, include it as an argument:
curl -X POST \
-H 'Content-Type: application/json' \
-d '{"key": "yu0tX54BcDAIuBp8RkNoldtcir9Dwip8",
"limit": 30,
"labels": ["Bestsellers"]
"visitor": null}' \
http://api.clerk.io/v2/recommendations/popular
If you are have questions regarding visitor tracking, please don’t hesitate reaching out to our Customer Success team through the live-chat in the lower right corner.